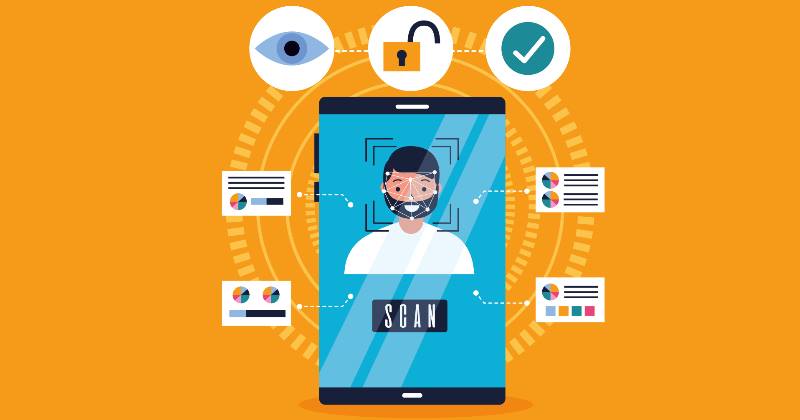
Sometimes you have a need to call an Azure Function with Azure AD enabled authentication from Logic App or from another Function or Azure Web App. For example, you have an API for your SPFx solution and also have a requirement to build a Logic App, which uses some methods from the web API. In that case, you should somehow perform the authentication to call your Azure AD protected function. You can easily solve this problem with Managed Identity.
Let's see how it works in practice.
NOTE. Currently, this method works only for Logic Apps and not for Power Automate. Simply because Logic Apps are configured in Azure, thus they have corresponding interface settings and authentication methods. Maybe it will change in the future.
Azure Function with Azure AD authentication
Assuming that you have such a function, you should also have a Client (Application) Id from an app registration in Azure AD. We need that Client Id in order to perform authentication using Managed Identity. Actually, that's all we need (and love, of course 🐞).
Creating and configuring Logic App
In your Azure Logic App, you should enable System assigned managed Identity:
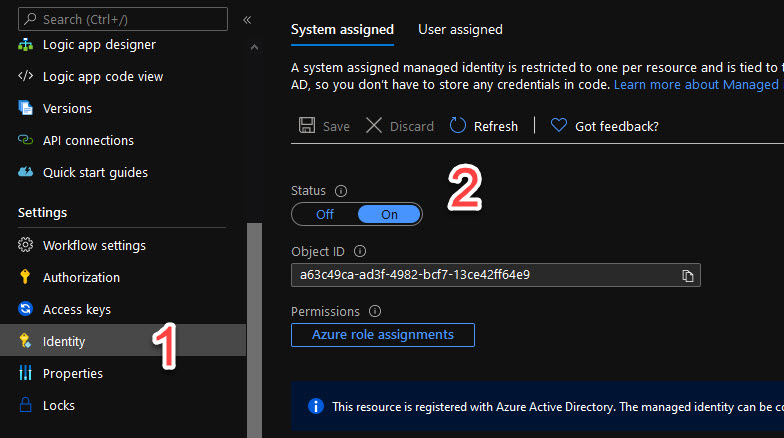
By doing this you register your Logic App as a separate active directory object, which can be used to generate access tokens to other Azure AD-protected resources.
Go to the designer view and add a new HTTP step. At the bottom select "Add new parameter" and then select "Authentication":
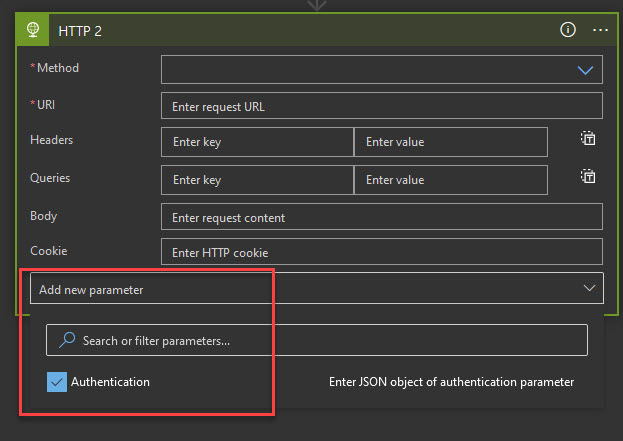
For authentication type select "Managed Identity", System-assigned. For the audience field use the Client Id from your app registration saved before.
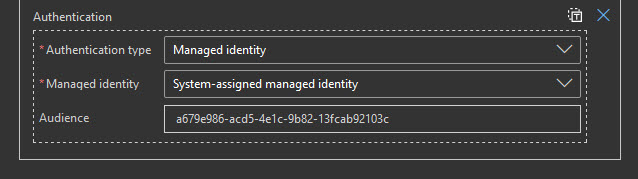
Now fill in the URI, Method and you're good to go! You don't have to manage any secrets, nor deal with low-level authentication. Instead, Azure handles everything under the hood.
The logic app will generate a new access token for the selected Client Id and will attach it to the Authorization header automatically so that your HTTP request will be authenticated.
How to call from another Function or Web app
You're not limited to using this approach with Logic apps only. Actually, managed identity can be used with many Azure services, which support Azure AD authentication.
For example, you can call the Azure AD-protected function securely from another function without managing any secrets or authentication. Instead, you can use Managed Identity.
To configure it, for your "caller" Azure Function you should also enable system-assigned Identity exactly the same as for Logic Apps above.
Below is the code needed to call Azure AD Function securely with Managed Identity:
var url = "https://<api url>";
var creds = new DefaultAzureCredential();
var token = await creds.GetTokenAsync(new Azure.Core.TokenRequestContext(new[] { "a679e986-acd5-4e1c-9b82-13fcab92103c" }));
using (HttpClient client = new HttpClient())
{
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", token.Token);
var result = await client.GetAsync(url);
var responseMessage = await result.Content.ReadAsStringAsync();
return new OkObjectResult(responseMessage);
}
How it works?
DefaultAzureCredential is just a container for different authentication methods. It iterates over all methods and tries to use first, which contains all needed credentials information. ManagedIdentityCredential is one authentication classes used by DefaultAzureCredential. It also means that you can use the ManagedIdentityCredential object directly, but DefaultAzureCredential just provides some convenience when debugging locally.
Under the hood, ManagedIdentityCredential checks whether managed identity is enabled. If yes, it uses managed identity flow to perform an authentication. The guid in the GetTokenAsync is a Client Id from the Azure AD protected Function. As soon as you have an access token, you can easily call your protected resource.
By leveraging managed identity we can get rid of boilerplate code for token generation and different secrets in code.
Title image attribution - Technology vector created by gstudioimagen - www.freepik.com